誠邀您參加全球知名外匯經紀商OANDA的自營交易(Prop Trader)
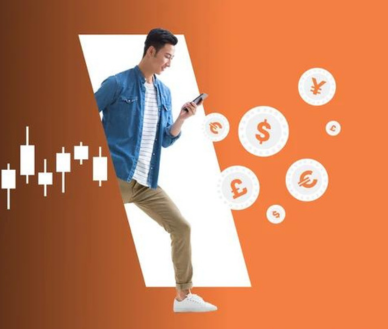
報名OANDA自營交易(Prop Trader),並通過我們的考核,您就可以使用OANDA提供的資金進行交易,獲得高達80%的交易利潤分成。
優化了挑戰塞交易規則
無最低交易天數限制等優化了挑戰賽的交易規則。
500,000美元交易資金
您可以使用最高500,000美元的資金進行交易。
豐富的交易商品
您可以交易包括外匯、黃金、原油、股票指數等多種商品。
在不同的時間軸圖表中 同步滑鼠游標的方法解析
設定全域變數的「Set」
在「MQL程式語言的全域變數說明」文章中,已經完成了顯示十字線的步驟;本次會將游標位置暫時保存於全域變數中,再將儲存的數值以「OnTimer」進行讀取,並修改顯示的編碼。如此一來,在並列顯示不同的時間週期圖表時,將能夠同步滑鼠游標(十字線)的動作。參考文章:MQL程式語言的全域變數說明
本次使用的是全域變數的「Set」。選擇MQL4相關參考目錄中的「Global Variables of the Terminal」→「GlobalVariableSet」即可確認參數。本次需設定變數的名稱與數值;名稱為「GVlauePrice」、數值則為「price」與「time」。
OnChartEvent下方將添加下列兩項公式。
GlobalVariableSet(“GVlauePrice”, price);
GlobalVariableSet(“GVlaueTime”, time);
GlobalVariableSet的設定項目 | |
name | 設定全域變數的名稱 |
value | 設定數值 |
從Timer函數中調出全域變數
透過GlobalVariableSet將全域變數從Timer函數中調出。為了使用計時器,需宣告計時器運作的間隔秒數,故應在OnInit下方添加「EventSetMillisecondTimer(100);」。由於此處的單位為千分之一秒的「Millisecond」,因此若設定為100千分之一秒,計時器就會間隔0.1秒運作。同時,也應一併定義OnDeinit下方的計時器取消項目「EventKillTimer();」。接下來,本次將運用以「Get」叫出的全域變數數值。Get的參數可從MQL4相關參考的「Global Variables of the Terminal」→「GlobalVariableGet」加以確認。OnTimer下方則如以下所示進行設定。由於變數本身為浮動的小數點,因此需注意寫入並變更「(datetime)」的型態。
double price = GlobalVariableGet(“GVlauePrice”);如此即為完成。進行編譯並整理兩者的圖表系列,將會看見十字線同步運作。
datetime time = (datetime)GlobalVariableGet(“GVlaueTime”);
本次介紹的屬於較特殊的使用方式,例如「將指標數值傳輸至全域變數,並以EA加以運用」等等;其他亦有應用型的方式可供選擇。
原始碼
本次製作的原始碼如以下所示。//+——————————————————————+
//| LineSync_demo.mq4 |
//| Copyright 2021, MetaQuotes Software Corp. |
//| https://www.mql5.com |
//+——————————————————————+
#property copyright “Copyright 2021, MetaQuotes Software Corp.”
#property link “https://www.mql5.com”
#property version “1.00”
#property strict
#property indicator_chart_window
//+——————————————————————+
//| Custom indicator initialization function |
//+——————————————————————+
int OnInit()
{
//— indicator buffers mapping
ChartSetInteger(0, CHART_EVENT_MOUSE_MOVE, true);
EventSetMillisecondTimer(100);
//—
return(INIT_SUCCEEDED);
}
//+——————————————————————+
//| Custom indicator deinit function |
//+——————————————————————+
void OnDeinit(const int reason)
{
ObjectDelete(0, “VLine”);
ObjectDelete(0, “HLine”);
EventKillTimer();
}
//+——————————————————————+
//| Custom indicator iteration function |
//+——————————————————————+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
//—
//— return value of prev_calculated for next call
return(rates_total);
}
//+——————————————————————+
//| Timer function |
//+——————————————————————+
void OnTimer()
{
//—
double price = GlobalVariableGet(“GVlauePrice”);
datetime time = (datetime)GlobalVariableGet(“GVlaueTime”);
HLineCreate(0, “HLine”, 0, price);
VLineCreate(0, “VLine”, 0, time);
}
//+——————————————————————+
//| ChartEvent function |
//+——————————————————————+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
//—
if(id == CHARTEVENT_MOUSE_MOVE) {
int x = (int)lparam;
int y = (int)dparam;
int win = 0;
double price;
datetime time;
if (ChartXYToTimePrice(0, x, y, win, time, price)) {
GlobalVariableSet(“GVlauePrice”, price);
GlobalVariableSet(“GVlaueTime”, time);
}
}
}
//+——————————————————————+
//| Create the vertical line |
//+——————————————————————+
bool VLineCreate(const long chart_ID = 0, // chart’s ID
const string name = “VLine”, // line name
const int sub_window = 0, // subwindow index
datetime time = 0, // line time
const color clr = clrWhite, // line color
const ENUM_LINE_STYLE style = STYLE_SOLID, // line style
const int width = 1, // line width
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
{
//— if the line time is not set, draw it via the last bar
if(!time)
time = TimeCurrent();
//— reset the error value
ResetLastError();
//— create a vertical line
if(!ObjectCreate(chart_ID, name, OBJ_VLINE, sub_window, time, 0)) {
/* Print(__FUNCTION__,
“: failed to create a vertical line! Error code = “,GetLastError()); */
ObjectSetInteger(chart_ID, name, OBJPROP_TIME, 0, time);
return(false);
}
//— set line color
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
//— set line display style
ObjectSetInteger(chart_ID, name, OBJPROP_STYLE, style);
//— set line width
ObjectSetInteger(chart_ID, name, OBJPROP_WIDTH, width);
//— display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
//— enable (true) or disable (false) the mode of moving the line by mouse
//— when creating a graphical object using ObjectCreate function, the object cannot be
//— highlighted and moved by default. Inside this method, selection parameter
//— is true by default making it possible to highlight and move the object
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
//— hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
//— set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
ObjectSetString(chart_ID, name, OBJPROP_TOOLTIP, “\n”);
//— successful execution
return(true);
}
//+——————————————————————+
//| Create the horizontal line |
//+——————————————————————+
bool HLineCreate(const long chart_ID = 0, // chart’s ID
const string name = “HLine”, // line name
const int sub_window = 0, // subwindow index
double price = 0, // line price
const color clr = clrWhite, // line color
const ENUM_LINE_STYLE style = STYLE_SOLID, // line style
const int width = 1, // line width
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
{
//— if the price is not set, set it at the current Bid price level
if(!price)
price = SymbolInfoDouble(Symbol(), SYMBOL_BID);
//— reset the error value
ResetLastError();
//— create a horizontal line
if(!ObjectCreate(chart_ID, name, OBJ_HLINE, sub_window, 0, price)) {
/* Print(__FUNCTION__,
“: failed to create a horizontal line! Error code = “,GetLastError());*/
ObjectSetDouble(chart_ID, name, OBJPROP_PRICE, 0, price);
return(false);
}
//— set line color
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
//— set line display style
ObjectSetInteger(chart_ID, name, OBJPROP_STYLE, style);
//— set line width
ObjectSetInteger(chart_ID, name, OBJPROP_WIDTH, width);
//— display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
//— enable (true) or disable (false) the mode of moving the line by mouse
//— when creating a graphical object using ObjectCreate function, the object cannot be
//— highlighted and moved by default. Inside this method, selection parameter
//— is true by default making it possible to highlight and move the object
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
//— hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
//— set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
ObjectSetString(chart_ID, name, OBJPROP_TOOLTIP, “\n”);
//— successful execution
return(true);
}
//+——————————————————————+
將EA自動程式交易應用於外匯與差價合約交易中
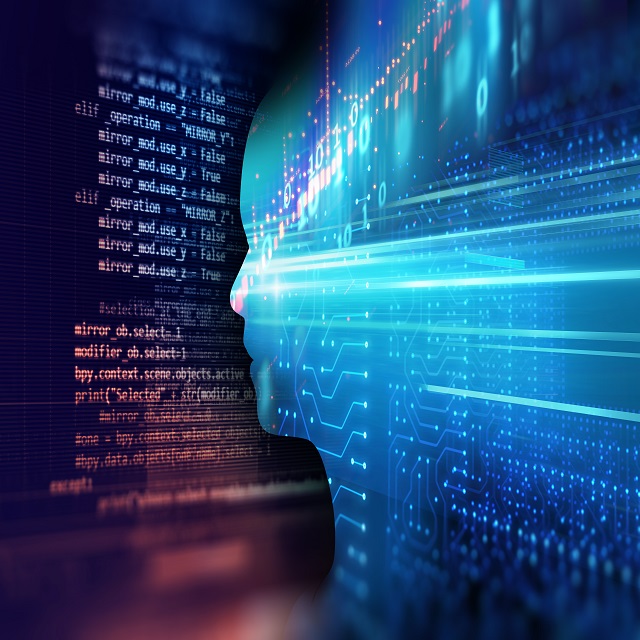
我們以圖文形式詳細介紹有關EA自動程式交易的基本知識,以及在MT4/MT5平台上的安裝、參數設定方法、編碼等等內容。另外,對持有OANDA帳戶的客戶,還可以免費使用我們的獨有EA與指標工具。
誠邀您參加全球知名外匯經紀商OANDA的自營交易(Prop Trader)
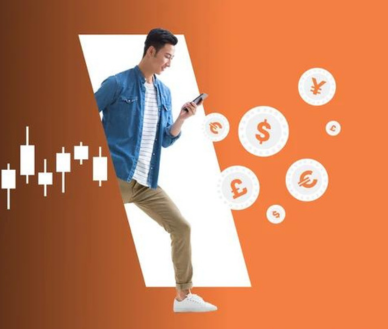
報名OANDA自營交易(Prop Trader),並通過我們的考核,您就可以使用OANDA提供的資金進行交易,獲得高達80%的交易利潤分成。
優化了挑戰塞交易規則
無最低交易天數限制等優化了挑戰賽的交易規則。
500,000美元交易資金
您可以使用最高500,000美元的資金進行交易。
豐富的交易商品
您可以交易包括外匯、黃金、原油、股票指數等多種商品。